constraints
Module¶
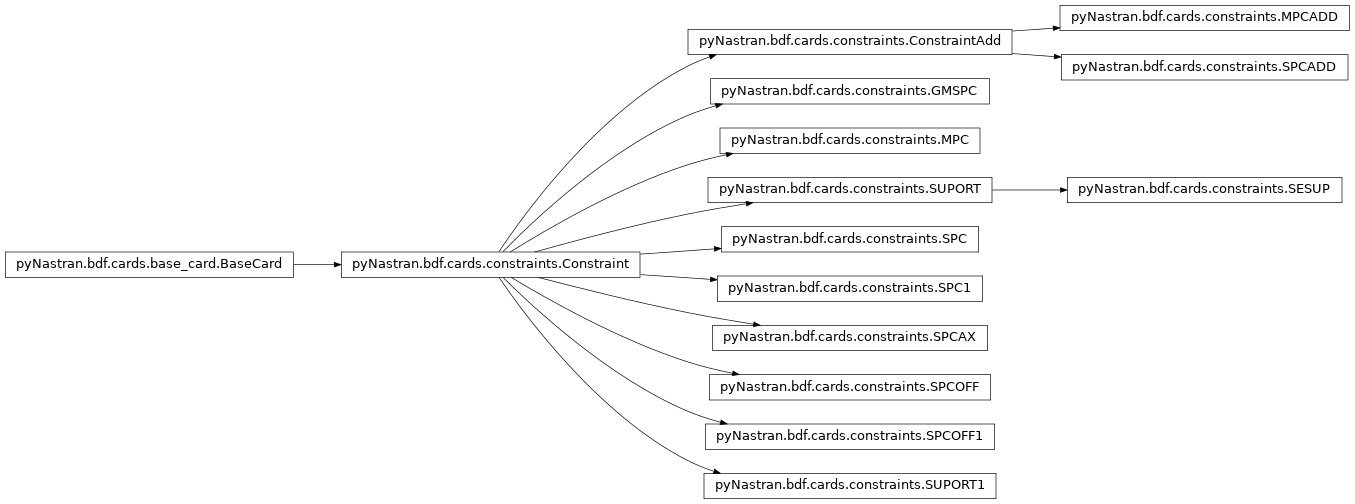
All constraint cards are defined in this file. This includes:
- Constraint
- SUPORT
- SUPORT1
- SPC
- SPC1
- SPCAX
- MPC
- GMSPC
- ConstraintADD
- SPCADD
- MPCADD
The ConstraintObject contain multiple constraints.
-
class
pyNastran.bdf.cards.constraints.
Constraint
[source]¶ Bases:
pyNastran.bdf.cards.base_card.BaseCard
- common class for:
- SUPORT / SUPORT1 / SESUP
- GMSPC
- MPC
- SPC / SPC1
- SPCAX
- SPCOFF / SPCOFF1
-
class
pyNastran.bdf.cards.constraints.
ConstraintAdd
[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
common class for SPCADD, MPCADD
-
class
pyNastran.bdf.cards.constraints.
GMSPC
(conid, component, entity, entity_id, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
-
classmethod
add_card
(card, comment='')[source]¶ Adds a GMSPC card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
type
= 'GMSPC'¶
-
classmethod
-
class
pyNastran.bdf.cards.constraints.
MPC
(conid, nodes, components, coefficients, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
Multipoint Constraint Defines a multipoint constraint equation of the form:
sum(A_j * u_j) = 0- where:
- uj represents degree-of-freedom Cj at grid or scalar point Gj. Aj represents the scale factor
1 2 3 4 5 6 7 8 9 MPC SID G1 C1 A1 G2 C2 A2 G3 C3 A3 … Creates an MPC card
Parameters: - conid : int
Case Control MPC id
- nodes : List[int]
GRID/SPOINT ids
- components : List[str]
the degree of freedoms to constrain (e.g., ‘1’, ‘123’)
- coefficients : List[float]
the scaling coefficients
-
_properties
= ['node_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds an MPC card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
coefficients
= None¶ Coefficient. (Real; Default = 0.0 except A1 must be nonzero.)
-
components
= None¶ Component number. (Any one of the Integers 1 through 6 for grid points; blank or zero for scalar points.)
-
conid
= None¶ Set identification number. (Integer > 0)
-
constraints
¶
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
enforced
¶
-
gids
¶
-
gids_ref
¶
-
node_ids
¶
-
nodes
= None¶ Identification number of grid or scalar point. (Integer > 0)
-
object_attributes
(self, mode='public', keys_to_skip=None, filter_properties=False)[source]¶ See also
pyNastran.utils.object_attributes(…)
-
object_methods
(self, mode='public', keys_to_skip=None)[source]¶ See also
pyNastran.utils.object_methods(…)
-
type
= 'MPC'¶
-
class
pyNastran.bdf.cards.constraints.
MPCADD
(conid, sets, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.ConstraintAdd
Defines a multipoint constraint equation of the form \(\Sigma_j A_j u_j =0\) where \(u_j\) represents degree-of-freedom \(C_j\) at grid or scalar point \(G_j\).
1 2 3 4 MPCADD 2 1 3 -
_properties
= ['ids', 'mpc_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MPCADD card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
ids
¶
-
mpc_ids
¶
-
type
= 'MPCADD'¶
-
-
class
pyNastran.bdf.cards.constraints.
SESUP
(nodes, Cs, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.SUPORT
-
type
= 'SESUP'¶
-
-
class
pyNastran.bdf.cards.constraints.
SPC
(conid, nodes, components, enforced, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
Defines enforced displacement/temperature (static analysis) velocity/acceleration (dynamic analysis).
1 2 3 4 5 6 7 8 SPC SID G1 C1 D1 G2 C2 D2 SPC 2 32 3 -2.6 5 Creates an SPC card, which defines the degree of freedoms to be constrained
Parameters: - conid : int
constraint id
- nodes : List[int]
GRID/SPOINT ids
- components : List[str]
the degree of freedoms to constrain (e.g., ‘1’, ‘123’)
- enforced : List[float]
the constrained value for the given node (typically 0.0)
- comment : str; default=’‘
a comment for the card
- .. note:: len(nodes) == len(components) == len(enforced)
- .. warning:: non-zero enforced deflection requires an SPCD as well
-
_properties
= ['node_ids', 'constraints', 'gids_ref', 'gids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds an SPC card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
constraints
¶
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
gids
¶
-
gids_ref
¶
-
node_ids
¶
-
object_attributes
(self, mode='public', keys_to_skip=None, filter_properties=False)[source]¶ See also
pyNastran.utils.object_attributes(…)
-
object_methods
(self, mode='public', keys_to_skip=None)[source]¶ See also
pyNastran.utils.object_methods(…)
-
type
= 'SPC'¶
-
class
pyNastran.bdf.cards.constraints.
SPC1
(conid, components, nodes, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
1 2 3 4 5 6 7 8 9 SPC1 SID C G1 G2 G3 G4 G5 G6 G7 G8 G9 etc. SPC1 3 246 209075 209096 209512 209513 209516 SPC1 3 2 1 3 10 9 6 5 2 8 SPC1 SID C G1 THRU G2 SPC1 313 12456 6 THRU 32 Creates an SPC1 card, which defines the degree of freedoms to be constrained to a value of 0.0
Parameters: - conid : int
constraint id
- components : str
the degree of freedoms to constrain (e.g., ‘1’, ‘123’)
- nodes : List[int]
GRID/SPOINT ids
- comment : str; default=’‘
a comment for the card
-
_properties
= ['node_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a SPC1 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
constraints
¶
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
node_ids
¶
-
type
= 'SPC1'¶
-
class
pyNastran.bdf.cards.constraints.
SPCADD
(conid, sets, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.ConstraintAdd
Defines a single-point constraint set as a union of single-point constraint sets defined on SPC or SPC1 entries.
1 2 3 4 SPCADD 2 1 3 -
_properties
= ['ids', 'spc_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a SPCADD card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
ids
¶
-
spc_ids
¶
-
type
= 'SPCADD'¶
-
-
class
pyNastran.bdf.cards.constraints.
SPCAX
(conid, ringax, hid, component, enforced, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
Defines a set of single-point constraints or enforced displacements for conical shell coordinates.
1 2 3 4 5 6 SPCAX SID RID HID C D SPCAX 2 3 4 13 6.0 Creates an SPCAX card
Parameters: - conid : int
Identification number of a single-point constraint set.
- ringax : int
Ring identification number. See RINGAX entry.
- hid : int
Harmonic identification number. (Integer >= 0)
- component : int
Component identification number. (Any unique combination of the Integers 1 through 6.)
- enforced : float
Enforced displacement value
-
classmethod
add_card
(card, comment='')[source]¶ Adds a SPCAX card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
component
= None¶ Component identification number. (Any unique combination of the Integers 1 through 6.)
-
conid
= None¶ Identification number of a single-point constraint set.
-
enforced
= None¶ Enforced displacement value
-
hid
= None¶ Harmonic identification number. (Integer >= 0)
-
ringax
= None¶ Ring identification number. See RINGAX entry.
-
type
= 'SPCAX'¶
-
class
pyNastran.bdf.cards.constraints.
SPCOFF
(nodes, components, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
1 2 3 4 5 6 7 8 9 SPC G1 C1 G2 C2 G3 C3 G4 C4 SPC 32 3 5 -
classmethod
add_card
(card, comment='')[source]¶ Adds a SPCOFF card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
constraints
¶
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
node_ids
¶
-
type
= 'SPCOFF'¶
-
classmethod
-
class
pyNastran.bdf.cards.constraints.
SPCOFF1
(components, nodes, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
-
classmethod
add_card
(card, comment='')[source]¶ Adds a SPCOFF1 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
constraints
¶
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
node_ids
¶
-
type
= 'SPCOFF1'¶
-
classmethod
-
class
pyNastran.bdf.cards.constraints.
SUPORT
(nodes, Cs, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
Defines determinate reaction degrees-of-freedom in a free body.
1 2 3 4 5 6 7 8 9 SUPORT ID1 C1 ID2 C2 ID3 C3 ID4 C4 Creates a SUPORT card, which defines free-body reaction points. This is always active.
Parameters: - nodes : List[int]
the nodes to release
- Cs : List[str]
compoents to support at each node
- comment : str; default=’‘
a comment for the card
-
_properties
= ['node_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a SUPORT card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
node_ids
¶
-
type
= 'SUPORT'¶
-
class
pyNastran.bdf.cards.constraints.
SUPORT1
(conid, nodes, Cs, comment='')[source]¶ Bases:
pyNastran.bdf.cards.constraints.Constraint
Defines determinate reaction degrees-of-freedom (r-set) in a free body-analysis. SUPORT1 must be requested by the SUPORT1 Case Control command.
1 2 3 4 5 6 7 8 SUPORT1 SID ID1 C1 ID2 C2 ID3 C3 SUPORT1 1 2 23 4 15 5 0 Creates a SUPORT card, which defines free-body reaction points.
Parameters: - conid : int
Case Control SUPORT id
- nodes : List[int]
the nodes to release
- Cs : List[str]
compoents to support at each node
- comment : str; default=’‘
a comment for the card
-
_properties
= ['node_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a SUPORT1 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
node_ids
¶
-
type
= 'SUPORT1'¶