materials
Module¶
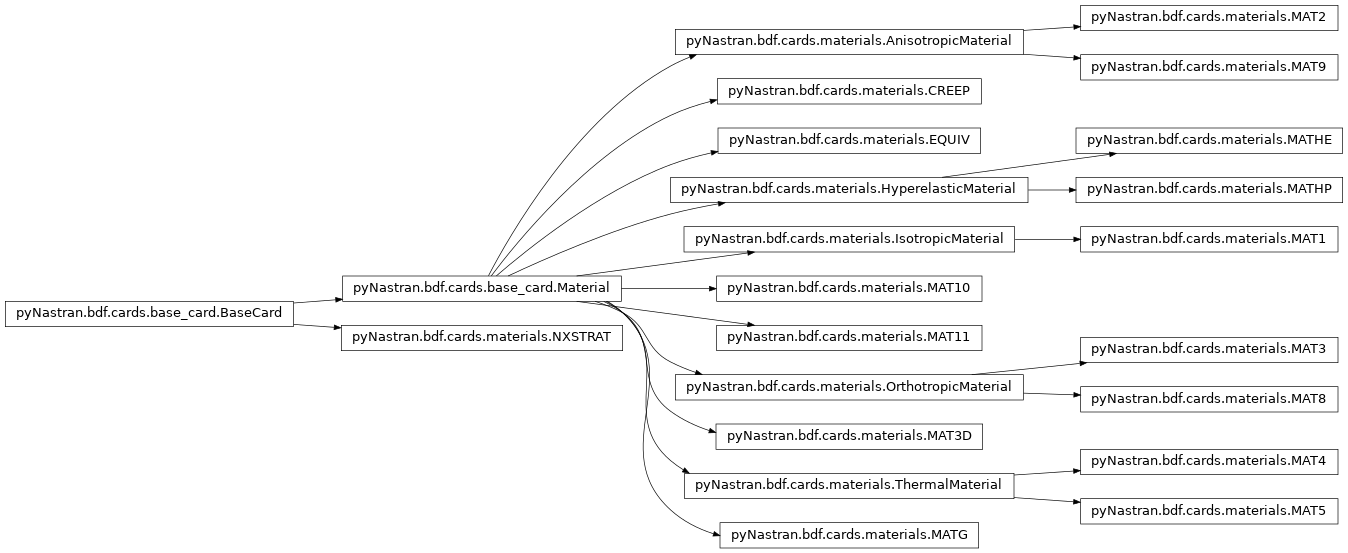
All material cards are defined in this file. This includes:
- CREEP
- MAT1 (isotropic solid/shell)
- MAT2 (anisotropic)
- MAT3 (linear orthotropic)
- MAT4 (thermal)
- MAT5 (thermal)
- MAT8 (orthotropic shell)
- MAT9 (anisotropic solid)
- MAT10 (fluid element)
- MATHP (hyperelastic)
- MATHE (hyperelastic)
All cards are Material objects.
-
class
pyNastran.bdf.cards.materials.
AnisotropicMaterial
[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
Anisotropic Material Class
-
class
pyNastran.bdf.cards.materials.
CREEP
(mid, T0, exp, form, tidkp, tidcp, tidcs, thresh, Type, a, b, c, d, e, f, g, comment='')[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
-
classmethod
add_card
(card, comment='')[source]¶ Adds a CREEP card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'CREEP'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
EQUIV
(mid, field2, field3, field4, field5, field6, field7, comment='')[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
-
classmethod
add_card
(card, comment='')[source]¶ Adds an EQUIV card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
mid
= None¶ Identification number of a MAT1, MAT2, or MAT9 entry.
-
type
= 'EQUIV'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
HyperelasticMaterial
[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
Hyperelastic Material Class
-
class
pyNastran.bdf.cards.materials.
IsotropicMaterial
[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
Isotropic Material Class
-
class
pyNastran.bdf.cards.materials.
MAT1
(mid, E, G, nu, rho=0.0, a=0.0, tref=0.0, ge=0.0, St=0.0, Sc=0.0, Ss=0.0, mcsid=0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.IsotropicMaterial
Defines the material properties for linear isotropic materials.
1 2 3 4 5 6 7 8 9 MAT1 MID E G NU RHO A TREF GE ST SC SS MCSID Creates a MAT1 card
Parameters: - mid : int
material id
- E : float / None
Young’s modulus
- G : float / None
Shear modulus
- nu : float / None
Poisson’s ratio
- rho : float; default=0.
density
- a : float; default=0.
coefficient of thermal expansion
- tref : float; default=0.
reference temperature
- ge : float; default=0.
damping coefficient
- St / Sc / Ss : float; default=0.
tensile / compression / shear allowable
- mcsid : int; default=0
material coordinate system id used by PARAM,CURV
- comment : str; default=’‘
a comment for the card
- If E, G, or nu is None (only 1), it will be calculated
-
_properties
= ['_field_map', 'mp_name_map']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT1 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
mp_name_map
= {'A': 'a', 'E': 'e', 'G': 'g', 'GE': 'ge', 'NU': 'nu', 'RHO': 'rho', 'SC': 'sc', 'SS': 'ss', 'ST': 'st', 'TREF': 'tref'}¶
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT1'¶
-
class
pyNastran.bdf.cards.materials.
MAT10
(mid, bulk=None, rho=None, c=None, ge=0.0, gamma=None, table_bulk=None, table_rho=None, table_ge=None, table_gamma=None, comment='')[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
Defines material properties for fluid elements in coupled fluid-structural analysis.
1 2 3 4 5 6 7 8 9 MAT10 MID BULK RHO C GE ALPHA per MSC 2016
1 2 3 4 5 6 7 8 9 MAT10 MID BULK RHO C GE GAMMA TID_BULK TID_RHO TID_GE TID_GAMMA per NX 10
..note :: alpha is called gamma
Creates a MAT10 card
Parameters: - mid : int
material id
- bulk : float; default=None
Bulk modulus
- rho : float; default=None
Density
- c : float; default=None
Speed of sound
- ge : float; default=0.
Damping
- gamma : float; default=None
NX : ratio of imaginary bulk modulus to real bulk modulus; default=0.0 MSC : normalized admittance coefficient for porous material
- table_bulk : int; default=None
TABLEDx entry defining bulk modulus vs. frequency None for MSC Nastran
- table_rho : int; default=None
TABLEDx entry defining rho vs. frequency None for MSC Nastran
- table_ge : int; default=None
TABLEDx entry defining ge vs. frequency None for MSC Nastran
- table_gamma : int; default=None
TABLEDx entry defining gamma vs. frequency None for MSC Nastran
- comment : str; default=’‘
a comment for the card
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT10 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
mp_name_map
= {'RHO': 'rho'}¶
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT10'¶
-
class
pyNastran.bdf.cards.materials.
MAT11
(mid, e1, e2, e3, nu12, nu13, nu23, g12, g13, g23, rho=0.0, a1=0.0, a2=0.0, a3=0.0, tref=0.0, ge=0.0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
Defines the material properties for a 3D orthotropic material for isoparametric solid elements.
1 2 3 4 5 6 7 8 9 MAT11 MID E1 E2 E3 NU12 NU13 NU23 G12 G13 G23 RHO A1 A2 A3 TREF GE -
_properties
= ['_field_map', 'mp_name_map']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT11 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
mp_name_map
= {'E1': 'e1', 'E2': 'e2', 'E3': 'e3'}¶
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT11'¶
-
-
class
pyNastran.bdf.cards.materials.
MAT2
(mid, G11, G12, G13, G22, G23, G33, rho=0.0, a1=None, a2=None, a3=None, tref=0.0, ge=0.0, St=None, Sc=None, Ss=None, mcsid=None, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.AnisotropicMaterial
Defines the material properties for linear anisotropic materials for two-dimensional elements.
1 2 3 4 5 6 7 8 9 MAT2 MID G11 G12 G13 G22 G23 G33 RHO A1 A2 A3 TREF GE ST SC SS MCSID -
_properties
= ['_field_map', 'mp_name_map']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT2 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
mp_name_map
= {'A1': 'a1', 'A2': 'a2', 'A3': 'a3', 'G11': 'G11', 'G12': 'G12', 'G13': 'G13', 'G22': 'G22', 'G23': 'G23', 'G33': 'G33', 'RHO': 'rho', 'TREF': 'tref'}¶
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT2'¶
-
-
class
pyNastran.bdf.cards.materials.
MAT3
(mid, ex, eth, ez, nuxth, nuthz, nuzx, rho=0.0, gzx=None, ax=0.0, ath=0.0, az=0.0, tref=0.0, ge=0.0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.OrthotropicMaterial
Defines the material properties for linear orthotropic materials used by the CTRIAX6 element entry.
1 2 3 4 5 6 7 8 9 MAT3 MID EX ETH EZ NUXTH NUTHZ NUZX RHO GZX AX ATH AZ TREF GE -
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT3 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT3'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
MAT3D
(mid, e1, e2, e3, nu12, nu13, nu23, g12, g13, g23, rho=0.0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
Defines the material properties for a 3D orthotropic material for isoparametric solid elements.
1 2 3 4 5 6 7 8 9 MAT3D MID E1 E2 E3 G12 G13 G23 NU12 NU12 NU13 NU23 RHO This is a VABS specific card that is almost identical to the MAT11.
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT3D card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT3D'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
MAT4
(mid, k, cp=0.0, rho=1.0, H=None, mu=None, hgen=1.0, ref_enthalpy=None, tch=None, tdelta=None, qlat=None, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.ThermalMaterial
Defines the constant or temperature-dependent thermal material properties for conductivity, heat capacity, density, dynamic viscosity, heat generation, reference enthalpy, and latent heat associated with a single-phase change.
1 2 3 4 5 6 7 8 9 MAT4 MID K CP RHO MU H HGEN REFENTH TCH TDELTA QLAT -
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT4 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'MAT4'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
MAT5
(mid, kxx=0.0, kxy=0.0, kxz=0.0, kyy=0.0, kyz=0.0, kzz=0.0, cp=0.0, rho=1.0, hgen=1.0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.ThermalMaterial
Defines the thermal material properties for anisotropic materials.
1 2 3 4 5 6 7 8 9 MAT5 MID KXX KXY KXZ KYY KYZ KZZ CP RHO HGEN Creates a MAT5, which defines the thermal material properties for an anisotropic material
Parameters: - mid : int
material id
- kxx : float; default==0.
???
- kxy : float; default==0.
???
- kxz : float; default==0.
???
- kyy : float; default==0.
???
- kyz : float; default==0.
???
- kzz : float; default==0.
???
- cp : float; default==0.
???
- rho : float; default==1.
???
- hgen : float; default=1.
???
- comment : str; default=’‘
a comment for the card
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT5 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
mid
= None¶ Thermal conductivity (assumed default=0.0)
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT5'¶
-
class
pyNastran.bdf.cards.materials.
MAT8
(mid, e11, e22, nu12, g12=0.0, g1z=100000000.0, g2z=100000000.0, rho=0.0, a1=0.0, a2=0.0, tref=0.0, Xt=0.0, Xc=None, Yt=0.0, Yc=None, S=0.0, ge=0.0, F12=0.0, strn=0.0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.OrthotropicMaterial
Defines the material property for an orthotropic material for isoparametric shell elements.
1 2 3 4 5 6 7 8 9 MAT8 MID E1 E2 NU12 G12 G1Z G2Z RHO A1 A2 TREF Xt Xc Yt Yc S GE1 F12 STRN -
_properties
= ['_field_map', 'mp_name_map']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT8 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
mp_name_map
= {'A1': 'a1', 'A2': 'a2', 'E1': 'e11', 'E2': 'e22', 'G12': 'g12', 'G1Z': 'g1z', 'NU12': 'nu12', 'RHO': 'rho'}¶
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT8'¶
-
-
class
pyNastran.bdf.cards.materials.
MAT9
(mid, G11=0.0, G12=0.0, G13=0.0, G14=0.0, G15=0.0, G16=0.0, G22=0.0, G23=0.0, G24=0.0, G25=0.0, G26=0.0, G33=0.0, G34=0.0, G35=0.0, G36=0.0, G44=0.0, G45=0.0, G46=0.0, G55=0.0, G56=0.0, G66=0.0, rho=0.0, A=None, tref=0.0, ge=0.0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.AnisotropicMaterial
Defines the material properties for linear, temperature-independent, anisotropic materials for solid isoparametric elements
See also
PSOLID entry description
1 2 3 4 5 6 7 8 9 MAT9 MID G11 G12 G13 G14 G15 G16 G22 G23 G24 G25 G26 G33 G34 G35 G36 G44 G45 G46 G55 G56 G66 RHO A1 A2 A3 A4 A5 A6 TREF GE -
_properties
= ['_field_map', 'mp_name_map']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MAT9 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
mid
= None¶ Material ID
-
mp_name_map
= {'A1': 'A[0]', 'A2': 'A[1]', 'A3': 'A[2]', 'A4': 'A[3]', 'A5': 'A[4]', 'A6': 'A[5]', 'G11': 'G11', 'G12': 'G12', 'G13': 'G13', 'G14': 'G14', 'G15': 'G15', 'G16': 'G16', 'G22': 'G22', 'G23': 'G23', 'G24': 'G24', 'G25': 'G25', 'G26': 'G26', 'G33': 'G33', 'G34': 'G34', 'G35': 'G35', 'G36': 'G36', 'G44': 'G44', 'G45': 'G45', 'G46': 'G46', 'G55': 'G55', 'G56': 'G56', 'G66': 'G66', 'GE': 'ge', 'RHO': 'rho', 'TREF': 'tref'}¶
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MAT9'¶
-
-
class
pyNastran.bdf.cards.materials.
MATG
(mid, idmem, behav, tabld, tablu, yprs, epl, gpl, gap=0.0, tab_yprs=None, tab_epl=None, tab_gpl=None, tab_gap=None, comment='')[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
1 2 3 4 5 6 7 8 9 MATG MID IDMEM BEHAV TABLD TABLU1 TABLU2 TABLU3 TABLU4 TABLU5 TABLU6 TABLU7 TABLU8 TABLU9 TABLU10 YPRS EPL GPL GAP TABYPRS TABEPL TABGPL TABGAP per MSC 2016
1 2 3 4 5 6 7 8 9 MATG MID IDMEM BEHAV TABLD TABLU1 TABLU2 TABLU3 TABLU4 TABLU5 TABLU6 TABLU7 TABLU8 TABLU9 TABLU10 YPRS EPL GPL per NX 10
MATG 100 10 0 1001 1002 1003 0.0 -
classmethod
add_card
(card, comment='')[source]¶ Adds a MATG card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MATG'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
MATHE
(mid, model, bulk, rho, texp, mus, alphas, betas, mooney, sussbat, aboyce, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.HyperelasticMaterial
Creates a MATHE hyperelastic material
model = MOONEY (default)
1 2 3 4 5 6 7 MATHE MID Model K RHO TEXP C10 C01 C20 C11 C02 C30 C21 C12 C03 model (NX) = OGDEN, FOAM
1 2 3 4 5 6 7 MATHE MID Model K RHO TEXP MU1 | ALPHA1 BETA1 MU2 | ALPHA2 BETA2 MU3 ALPHA3 BETA3 MU4 | ALPHA4 BETA4 MU5 ALPHA5 BETA5 MU6 | ALPHA6 BETA6 MU7 ALPHA7 BETA7 MU8 | ALPHA8 BETA8 MU9 ALPHA9 BETA9 the last two lines are NX only lines
model (NX) = ABOYCE
1 2 3 4 5 6 7 MATHE MID Model K RHO TEXP NKT N1 D1 D2 D3 D4 D5 the last line is an MSC only line
model (NX) = SUSSBAT
1 2 3 4 5 6 7 MATHE MID Model K RHO TEXP TAB1 SSTYPE RELERR model (NX) = MOONEY (default)
1 2 3 4 5 6 7 8 9 MATHE MID Model K RHO TEXP TREF GE C10 C01 D1 TAB1 TAB2 TAB3 TAB4 TABD C20 C11 C02 D2 NA C30 C21 C12 C03 D3 C40 C31 C22 C13 C04 D4 C50 C41 C32 C23 C14 C05 D5 model (MSC) = OGDEN, FOAM
1 2 3 4 5 6 7 8 MATHE MID Model NOT K RHO TEXP MU1 ALPHA1 BETA1 MU2 ALPHA2 BETA2 MU3 ALPHA3 BETA3 MU4 ALPHA4 BETA4 MU5 ALPHA5 BETA5 D1 D2 D3 D4 D5 NOT is an MSC only parameter
the last line is an MSC only line
model (MSC) = ABOYCE, GENT
1 2 3 4 5 6 7 8 MATHE MID Model K RHO TEXP NKT N1 D1 D2 D3 D4 D5 the last line is an MSC only line
model (MSC) = GHEMi
1 2 3 4 5 6 7 8 MATHE MID Model K RHO Texp Tref GE MSC version
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MATHE card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MATHE'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
MATHP
(mid, a10=0.0, a01=0.0, d1=None, rho=0.0, av=0.0, tref=0.0, ge=0.0, na=1, nd=1, a20=0.0, a11=0.0, a02=0.0, d2=0.0, a30=0.0, a21=0.0, a12=0.0, a03=0.0, d3=0.0, a40=0.0, a31=0.0, a22=0.0, a13=0.0, a04=0.0, d4=0.0, a50=0.0, a41=0.0, a32=0.0, a23=0.0, a14=0.0, a05=0.0, d5=0.0, tab1=None, tab2=None, tab3=None, tab4=None, tabd=None, comment='')[source]¶ Bases:
pyNastran.bdf.cards.materials.HyperelasticMaterial
-
classmethod
add_card
(card, comment='')[source]¶ Adds a MATHP card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'MATHP'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
NXSTRAT
(sid, params, comment='')[source]¶ Bases:
pyNastran.bdf.cards.base_card.BaseCard
Strategy Parameters for SOLs 601 and 701
Defines parameters for solution control and strategy in advanced nonlinear structural analysis.
1 2 3 4 5 6 7 8 NXSTRAT ID Param1 Value1 Param2 Value2 Param3 Value3 Param4 Value4 Param5 Value5 etc NXSTRAT 1 AUTO 1 MAXITE 30 RTOL 0.005 ATSNEXT 3 -
classmethod
add_card
(card, comment='')[source]¶ Adds a NXSTRAT card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : [varies, …]
the fields that define the card
-
type
= 'NXSTRAT'¶
-
classmethod
-
class
pyNastran.bdf.cards.materials.
OrthotropicMaterial
[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
Orthotropic Material Class
-
class
pyNastran.bdf.cards.materials.
ThermalMaterial
[source]¶ Bases:
pyNastran.bdf.cards.base_card.Material
Thermal Material Class