optimization
Module¶
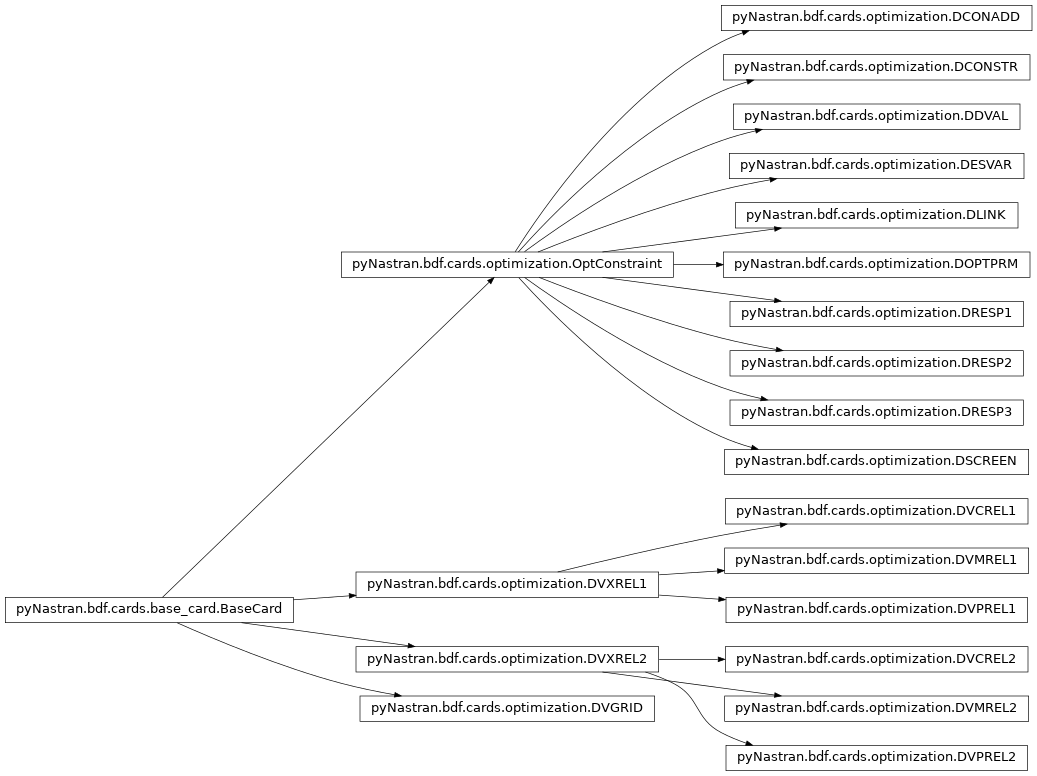
All optimization cards are defined in this file. This includes:
- dconstrs - DCONSTR
- dconadds - DCONADD
- ddvals - DDVAL
- dlinks - DLINK
- dresps - DRESP1, DRESP2, DRESP3
- dscreen - DSCREEN
- dvgrids - DVGRID
- desvars - DESVAR
- dvcrels - DVCREL1, DVCREL2
- dvmrels - DVMREL1, DVMREL2
- dvprels - DVPREL1, DVPREL2
- doptprm - DOPTPRM
some missing optimization flags http://mscnastrannovice.blogspot.com/2014/06/msc-nastran-design-optimization-quick.html
-
class
pyNastran.bdf.cards.optimization.
DCONADD
(oid, dconstrs, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
1 2 3 4 5 6 7 8 9 DCONADD DCID DC1 DC2 DC3 DC4 DC5 DC6 DC7 DC8 etc. DCONADD 10 4 12 -
classmethod
add_card
(card, comment='')[source]¶ Adds a DCONADD card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
dconstr_ids
¶
-
type
= 'DCONADD'¶
-
classmethod
-
class
pyNastran.bdf.cards.optimization.
DCONSTR
(oid, dresp_id, lid=-1e+20, uid=1e+20, lowfq=0.0, highfq=1e+20, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
1 2 3 4 5 6 7 DCONSTR DCID RID LALLOW/LID UALLOW/UID LOWFQ HIGHFQ DCONSTR 10 4 1.25 Creates a DCONSTR card
Parameters: - oid : int
unique optimization id
- dresp_id : int
DRESP1/2 id
- lid / uid=-1.e20 / 1.e20
lower/upper bound
- lowfq / highfq : float; default=0. / 1.e20
lower/upper end of the frequency range
- comment : str; default=’‘
a comment for the card
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DCONSTR card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'DCONSTR'¶
-
class
pyNastran.bdf.cards.optimization.
DDVAL
(oid, ddvals, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
1 2 3 4 5 6 7 8 9 DDVAL ID DVAL1 DVAL2 DVAL3 DVAL4 DVAL5 DVAL6 DVAL7 DDVAL ID DVAL1 THRU DVAL2 BY INC DDVAL 110 0.1 0.2 0.3 0.5 0.6 0.4 .7 THRU 1.0 BY 0.05 1.5 2.0 -
classmethod
add_card
(card, comment='')[source]¶ Adds a DDVAL card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
type
= 'DDVAL'¶
-
classmethod
-
class
pyNastran.bdf.cards.optimization.
DESVAR
(desvar_id, label, xinit, xlb=-1e+20, xub=1e+20, delx=None, ddval=None, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
Creates a DESVAR card
Parameters: - desvar_id : int
design variable id
- label : str
name of the design variable
- xinit : float
the starting point value for the variable
- xlb : float; default=-1.e20
the lower bound
- xub : float; default=1.e20
the lower bound
- delx : float; default=1.e20
fractional change allowed for design variables during approximate optimization NX if blank : take from DOPTPRM; otherwise 1.0 MSC if blank : take from DOPTPRM; otherwise 0.5
- ddval : int; default=None
- int : DDVAL id
allows you to set discrete values
None : continuous
- comment : str; default=’‘
a comment for the card
-
_properties
= ['value']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DESVAR card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
classmethod
export_to_hdf5
(h5_file, model, desvar_ids)[source]¶ exports the elements in a vectorized way
-
label
= None¶ user-defined name for printing purposes
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'DESVAR'¶ 1 2 3 4 5 6 7 8 DESVAR OID LABEL XINIT XLB XUB DELXV DDVAL
-
value
¶ gets the actual value for the DESVAR
-
class
pyNastran.bdf.cards.optimization.
DLINK
(oid, dependent_desvar, independent_desvars, coeffs, c0=0.0, cmult=1.0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
Multiple Design Variable Linking Relates one design variable to one or more other design variables.
1 2 3 4 5 6 7 8 9 DLINK ID DDVID C0 CMULT IDV1 C1 IDV2 C2 IDV3 C3 etc. Creates a DLINK card, which creates a variable that is a lienar ccombination of other design variables
Parameters: - oid : int
optimization id
- dependent_desvar : int
the DESVAR to link
- independent_desvars : List[int]
the DESVARs to combine
- coeffs : List[int]
the linear combination coefficients
- c0 : float; default=0.0
an offset
- cmult : float; default=1.0
an scale factor
- comment : str; default=’‘
a comment for the card
-
Ci
¶
-
IDv
¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DLINK card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
ddvid
¶
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'DLINK'¶
-
class
pyNastran.bdf.cards.optimization.
DOPTPRM
(params, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
causes a Nastran core dump if FSDMAX is nonzero and there is no stress case
Design Optimization Parameters Overrides default values of parameters used in design optimization
1 2 3 4 5 6 7 8 9 DOPTPRM PARAM1 VAL1 PARAM2 VAL2 PARAM3 VAL3 PARAM4 VAL4 PARAM5 VAL5 etc. -
classmethod
add_card
(card, comment='')[source]¶ Adds a DOPTPRM card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
defaults
= {'APRCOD': 2, 'AUTOSE': 0, 'CONV1': 0.001, 'CONV2': 1e-20, 'CONVDV': 0.001, 'CONVPR': 0.001, 'CT': -0.03, 'CTMIN': 0.003, 'DELB': 0.0001, 'DELP': 0.2, 'DELX': 0.5, 'DESMAX': 5, 'DISBEG': 0, 'DISCOD': 1, 'DLXESL': 0.5, 'DPMAX': 0.5, 'DPMIN': 0.01, 'DRATIO': 0.1, 'DSMXESL': 20, 'DXMAX': 1.0, 'DXMIN': 0.05, 'ETA1': 0.01, 'ETA2': 0.25, 'ETA3': 0.7, 'FSDALP': 0.9, 'FSDMAX': 0, 'GMAX': 0.005, 'GSCAL': 0.001, 'IGMAX': 0, 'IPRINT': 0, 'ISCAL': 0, 'METHOD': 0, 'NASPRO': 0, 'OBJMOD': 0, 'OPTCOD': 0, 'P1': 0, 'P2': 1, 'P2CALL': None, 'P2CBL': None, 'P2CC': None, 'P2CDDV': None, 'P2CM': None, 'P2CP': None, 'P2CR': None, 'P2RSET': None, 'PENAL': 0.0, 'PLVIOL': 0, 'PTOL': 1e+35, 'STPSCL': 1.0, 'TCHECK': -1, 'TDMIN': None, 'TREGION': 0, 'UPDFAC1': 2.0, 'UPDFAC2': 0.5}¶
-
type
= 'DOPTPRM'¶
-
classmethod
-
class
pyNastran.bdf.cards.optimization.
DRESP1
(dresp_id, label, response_type, property_type, region, atta, attb, atti, comment='', validate=False)[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
1 2 3 4 5 6 7 8 9 DRESP1 OID LABEL RTYPE PTYPE REGION ATTA ATTB ATTI DRESP1 103 STRESS2 STRESS PSHELL 9 3 DRESP1 1S1 CSTRAN3 CSTRAIN PCOMP 1 1 10000 Creates a DRESP1 card.
A DRESP1 is used to define a “simple” output result that may be optimized on. A simple result is a result like stress, strain, force, displacement, eigenvalue, etc. for a node/element that may be found in a non-optimization case.
Parameters: - dresp_id : int
response id
- lable : str
Name of the response
- response_type : str
Response type
- property_type : str
Element flag (PTYPE = ‘ELEM’), or property entry name, or panel flag for ERP responses (PTYPE = ‘PANEL’ - See Remark 34), or RANDPS ID. Blank for grid point responses. ‘ELEM’ or property name used only with element type responses (stress, strain, force, etc.) to identify the relevant element IDs, or the property type and relevant property IDs.
Must be {ELEM, PBAR, PSHELL, PCOMP, PANEL, etc.) PTYPE = RANDPS ID when RTYPE=PSDDISP, PSDVELO, or PSDACCL.
- region : int
Region identifier for constraint screening
- atta : int / float / str / blank
Response attribute
- attb : int / float / str / blank
Response attribute
- atti : List[int / float / str]
the response values to pull from List[int]:
list of grid ids list of property ids
- List[str]
‘ALL’
- comment : str; default=’‘
a comment for the card
- validate : bool; default=False
should the card be validated when it’s created
Examples
stress/PSHELL
>>> dresp_id = 103 >>> label = 'resp1' >>> response_type = 'STRESS' >>> property_type = 'PSHELL' >>> pid = 3 >>> atta = 9 # von mises upper surface stress >>> region = None >>> attb = None >>> atti = [pid] >>> DRESP1(dresp_id, label, response_type, property_type, region, atta, attb, atti)
stress/PCOMP
>>> dresp_id = 104 >>> label = 'resp2' >>> response_type = 'STRESS' >>> property_type = 'PCOMP' >>> pid = 3 >>> layer = 4 >>> atta = 9 # von mises upper surface stress >>> region = None >>> attb = layer >>> atti = [pid] >>> DRESP1(dresp_id, label, response_type, property_type, region, atta, attb, atti)
displacement - not done
>>> dresp_id = 105 >>> label = 'resp3' >>> response_type = 'DISP' >>> #atta = ??? >>> #region = ??? >>> #attb = ??? >>> atti = [nid] >>> DRESP1(dresp_id, label, response_type, property_type, region, atta, attb, atti)
not done >>> dresp_id = 105 >>> label = ‘resp3’ >>> response_type = ‘ELEM’ >>> #atta = ??? >>> #region = ??? >>> #attb = ??? >>> atti = [eid???] >>> DRESP1(dresp_id, label, response_type, property_type, region, atta, attb, atti)
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DRESP1 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
classmethod
export_to_hdf5
(h5_file, model, encoding)[source]¶ exports the dresps in a vectorized way
-
object_attributes
(self, mode='public', keys_to_skip=None, filter_properties=False)[source]¶ See also
pyNastran.utils.object_attributes(…)
-
ptype
¶
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
rtype
¶
-
safe_cross_reference
(self, model, xref_errors)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
type
= 'DRESP1'¶
-
class
pyNastran.bdf.cards.optimization.
DRESP2
(dresp_id, label, dequation, region, params, method='MIN', c1=1.0, c2=0.005, c3=10.0, comment='', validate=False)[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
Design Sensitivity Equation Response Quantities Defines equation responses that are used in the design, either as constraints or as an objective.
1 2 3 4 5 6 7 8 9 DRESP2 ID LABEL EQID/FUNC REGION METHOD C1 C2 C3 DESVAR DVID1 DVID2 DVID3 DVID4 DVID5 DVID6 DVID7 DVID8 etc. DTABLE LABL1 LABL2 LABL3 LABL4 LABL5 LABL6 LABL7 LABL8 etc. DRESP1 NR1 NR2 NR3 NR4 NR5 NR6 NR7 NR8 etc. DNODE G1 C1 G2 C2 G3 C3 G4 C4 etc. DVPREL1 DPIP1 DPIP2 DPIP3 DPIP4 DPIP5 DPIP6 DPIP7 DPIP8 DPIP9 etc. DVCREL1 DCIC1 DCIC2 DCIC3 DCIC4 DCIC5 DCIC6 DCIC7 DCIC8 DCIC9 etc. DVMREL1 DMIM1 DMIM2 DMIM3 DMIM4 DMIM5 DMIM6 DMIM7 DMIM8 DMIM9 etc. DVPREL2 DPI2P1 DPI2P2 DPI2P3 DPI2P4 DPI2P5 DPI2P6 DPI2P7 DPI2P8 DPI2P9 etc. DVCREL2 DCI2C1 DCI2C2 DCI2C3 DCI2C4 DCI2C5 DCI2C6 DCI2C7 DCI2C8 DCI2C9 etc. DVMREL2 DMI2M1 DMI2M2 DMI2M3 DMI2M4 DMI2M5 DMI2M6 DMI2M7 DMI2M8 DMI2M9 etc. DRESP2 NRR1 NRR2 NRR3 NRR4 NRR5 NRR6 NRR7 NRR8 etc. DVLREL1 DLIL1 DLIL2 DLIL3 DLIL4 DLIL5 DLIL6 DLIL7 DLIL8 etc. C1, C2, C3 are MSC specific
Creates a DRESP2 card.
A DRESP2 is used to define a “complex” output result that may be optimized on. A complex result is a result that uses:
- simple (DRESP1) results
- complex (DRESP2) results
- default values (DTABLE)
- DVCRELx values
- DVMRELx values
- DVPRELx values
- DESVAR values
- DNODE values
Then, an equation (DEQATN) is used to formulate an output response.
Parameters: - dresp_id : int
response id
- label : str
Name of the response
- dequation : int / str
int : DEQATN id str : an equation
- region : int
Region identifier for constraint screening
- params : dict[(index, card_type)] = values
the storage table for the response function index : int
a counter
- card_type : str
the type of card to pull from DESVAR, DVPREL1, DRESP2, etc.
- values : List[int]
the values for this response
- method : str; default=MIN
flag used for FUNC=BETA/MATCH FUNC = BETA
valid options are {MIN, MAX}
- FUNC = MATCH
valid options are {LS, BETA}
- c1 / c2 / c3 : float; default=1. / 0.005 / 10.0
constants for FUNC=BETA or FUNC=MATCH
- comment : str; default=’‘
a comment for the card
- validate : bool; default=False
should the card be validated when it’s created
- params = {
(0, ‘DRESP1’) = [10, 20], (1, ‘DESVAR’) = [30], (2, ‘DRESP1’) = [40],
- }
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DRESP2 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
classmethod
export_to_hdf5
(h5_file, model, encoding)[source]¶ exports the dresps in a vectorized way
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'DRESP2'¶
-
class
pyNastran.bdf.cards.optimization.
DRESP3
(dresp_id, label, group, Type, region, params, validate=False, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
1 2 3 4 5 6 7 8 9 DRESP3 ID LABEL GROUP TYPE REGION DESVAR DVID1 DVID2 DVID3 DVID4 DVID5 DVID6 DVID7 DVID8 etc. DTABLE LABL1 LABL2 LABL3 LABL4 LABL5 LABL6 LABL7 LABL8 etc. DRESP1 NR1 NR2 NR3 NR4 NR5 NR6 NR7 NR8 etc. DNODE G1 C1 G2 C2 G3 C3 G4 C4 etc. DVPREL1 DPIP1 DPIP2 DPIP3 DPIP4 DPIP5 DPIP6 DPIP7 DPIP8 DPIP9 etc. DVCREL1 DCIC1 DCIC2 DCIC3 DCIC4 DCIC5 DCIC6 DCIC7 DCIC8 DCIC9 etc. DVMREL1 DMIM1 DMIM2 DMIM3 DMIM4 DMIM5 DMIM6 DMIM7 DMIM8 DMIM9 etc. DVPREL2 DPI2P1 DPI2P2 DPI2P3 DPI2P4 DPI2P5 DPI2P6 DPI2P7 DPI2P8 DPI2P9 etc. DVCREL2 DCI2C1 DCI2C2 DCI2C3 DCI2C4 DCI2C5 DCI2C6 DCI2C7 DCI2C8 DCI2C9 etc. DVMREL2 DMI2M1 DMI2M2 DMI2M3 DMI2M4 DMI2M5 DMI2M6 DMI2M7 DMI2M8 DMI2M9 etc. DRESP2 NRR1 NRR2 NRR3 NRR4 NRR5 NRR6 NRR7 NRR8 etc. DVLREL1 DLIL1 DLIL2 DLIL3 DLIL4 DLIL5 DLIL6 DLIL7 DLIL8 etc. USRDATA String etc. Creates a DRESP3 card.
A DRESP3 is used to define a “complex” output result that may be optimized on. A complex result is a result that uses:
- simple (DRESP1) results
- complex (DRESP2) results
- default values (DTABLE)
- DVCRELx values
- DVMRELx values
- DVPRELx values
- DESVAR values
- DNODE values
- DVLREL1 values
- USRDATA
Then, an secondary code (USRDATA) is used to formulate an output response.
Parameters: - dresp_id : int
response id
- label : str
Name of the response
- group : str
Selects a specific external response routine
- Type : str
Refers to a specific user-created response calculation type in the external function evaluator
- region : str
Region identifier for constraint screening
- params : dict[(index, card_type)] = values
the storage table for the response function index : int
a counter
- card_type : str
the type of card to pull from DESVAR, DVPREL1, DRESP2, etc.
- values : List[int]
the values for this response
- comment : str; default=’‘
a comment for the card
- validate : bool; default=False
should the card be validated when it’s created
- params = {
(0, ‘DRESP1’) = [10, 20], (1, ‘DESVAR’) = [30], (2, ‘DRESP1’) = [40],
- }
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DRESP3 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
classmethod
export_to_hdf5
(h5_file, model, encoding)[source]¶ exports the dresps in a vectorized way
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'DRESP3'¶
-
class
pyNastran.bdf.cards.optimization.
DSCREEN
(rtype, trs=-0.5, nstr=20, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.OptConstraint
Creates a DSCREEN object
Parameters: - rtype : str
Response type for which the screening criteria apply
- trs : float
Truncation threshold
- nstr : int
Maximum number of constraints to be retained per region per load case
- comment : str; default=’‘
a comment for the card
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DSCREEN card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
nstr
= None¶ Maximum number of constraints to be retained per region per load case. (Integer > 0; Default = 20)
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
rtype
= None¶ Response type for which the screening criteria apply. (Character)
-
trs
= None¶ Truncation threshold. (Real; Default = -0.5)
-
type
= 'DSCREEN'¶
-
class
pyNastran.bdf.cards.optimization.
DVCREL1
(oid, element_type, eid, cp_name, dvids, coeffs, cp_min=None, cp_max=1e+20, c0=0.0, validate=False, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.DVXREL1
1 2 3 4 5 6 7 8 9 DVCREL1 ID TYPE EID CPNAME CPMIN CPMAX C0 DVID1 COEF1 DVID2 COEF2 DVID3 etc. DVCREL1 200000 CQUAD4 1 ZOFFS 1.0 200000 1.0 -
Type
¶
-
_properties
= ['desvar_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DVCREL1 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
object_attributes
(self, mode='public', keys_to_skip=None, filter_properties=False)[source]¶ See also
pyNastran.utils.object_attributes(…)
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'DVCREL1'¶
-
-
class
pyNastran.bdf.cards.optimization.
DVCREL2
(oid, element_type, eid, cp_name, deqation, dvids, labels, cp_min=None, cp_max=1e+20, validate=False, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.DVXREL2
1 2 3 4 5 6 7 8 9 DVCREL2 ID TYPE EID CPNAME/FID CPMIN CPMAX EQID DESVAR DVID1 DVID2 DVID3 DVID4 DVID5 DVID6 DVID7 DVID8 etc. DTABLE LABL1 LABL2 LABL3 LABL4 LABL5 LABL6 LABL7 LABL8 etc. -
Type
¶
-
_properties
= ['desvar_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DVCREL2 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
allowed_elements
= ['CQUAD4', 'CTRIA3', 'CBAR', 'CBEAM', 'CELAS1', 'CBUSH', 'CDAMP2']¶
-
calculate
(self, op2_model, subcase_id)[source]¶ this should really make a call the the DEQATN; see the PBEAM for an example of get/set_opt_value
-
cp_max
= None¶ Maximum value allowed for this property. (Real; Default = 1.0E20)
-
cp_min
= None¶ Minimum value allowed for this property. If CPNAME references a connectivity property that can only be positive, then the default value of CPMIN is 1.0E-15. Otherwise, it is -1.0E35. (Real) .. todo:: bad default (see DVCREL2)
-
cp_name
= None¶ Name of connectivity property, such as X1, X2, X3, ZOFFS, etc. (Character)
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
- .. todo:: add support for DEQATN cards to finish DVPREL2 xref
-
eid
= None¶ Element Identification number. (Integer > 0)
-
element_type
= None¶ Name of an element connectivity entry, such as CBAR, CQUAD4, etc. (Character)
-
object_attributes
(self, mode='public', keys_to_skip=None, filter_properties=False)[source]¶ See also
pyNastran.utils.object_attributes(…)
-
type
= 'DVCREL2'¶
-
-
class
pyNastran.bdf.cards.optimization.
DVGRID
(dvid, nid, dxyz, cid=0, coeff=1.0, comment='')[source]¶ Bases:
pyNastran.bdf.cards.base_card.BaseCard
1 2 3 4 5 6 7 8 DVGRID DVID GID CID COEFF N1 N2 N3 Creates a DVGRID card
Parameters: - dvid : int
DESVAR id
- nid : int
GRID/POINT id
- dxyz : (3, ) float ndarray
the amount to move the grid point
- cid : int; default=0
Coordinate system for dxyz
- coeff : float; default=1.0
the dxyz scale factor
- comment : str; default=’‘
a comment for the card
-
_properties
= ['desvar_id', 'node_id', 'coord_id']¶
-
static
add_card
(card, comment='')[source]¶ Adds a DVGRID card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
coord_id
¶
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
desvar_id
¶
-
node_id
¶
-
type
= 'DVGRID'¶
-
class
pyNastran.bdf.cards.optimization.
DVMREL1
(oid, mat_type, mid, mp_name, dvids, coeffs, mp_min=None, mp_max=1e+20, c0=0.0, validate=False, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.DVXREL1
Design Variable to Material Relation Defines the relation between a material property and design variables.
1 2 3 4 5 6 7 8 DVMREL1 ID TYPE MID MPNAME MPMIN MPMAX C0 DVID1 COEF1 DVID2 COEF2 DVID3 COEF3 etc. Creates a DVMREL1 card
Parameters: - oid : int
optimization id
- prop_type : str
material card name (e.g., MAT1)
- mid : int
material id
- mp_name : str
optimization parameter as a pname (material name; E)
- dvids : List[int]
DESVAR ids
- coeffs : List[float]
scale factors for DESVAR ids
- mp_min : float; default=None
minimum material property value
- mp_max : float; default=1e20
maximum material property value
- c0 : float; default=0.
offset factor for the variable
- validate : bool; default=False
should the variable be validated
- comment : str; default=’‘
a comment for the card
-
_properties
= ['desvar_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DVMREL1 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
object_attributes
(self, mode='public', keys_to_skip=None, filter_properties=False)[source]¶ See also
pyNastran.utils.object_attributes(…)
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'DVMREL1'¶
-
class
pyNastran.bdf.cards.optimization.
DVMREL2
(oid, mat_type, mid, mp_name, deqation, dvids, labels, mp_min=None, mp_max=1e+20, validate=False, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.DVXREL2
1 2 3 4 5 6 7 8 9 DVMREL2 ID TYPE MID MPNAME MPMIN MPMAX EQID DESVAR DVID1 DVID2 DVID3 DVID4 DVID5 DVID6 DVID7 DVID8 etc. DTABLE LABL1 LABL2 LABL3 LABL4 LABL5 LABL6 LABL7 LABL8 etc. Creates a DVMREL2 card
Parameters: - oid : int
optimization id
- mat_type : str
material card name (e.g., MAT1)
- mid : int
material id
- mp_name : str
optimization parameter as a pname (material name; E)
- deqation : int
DEQATN id
- dvids : List[int]; default=None
DESVAR ids
- labels : List[str]; default=None
DTABLE names
- mp_min : float; default=None
minimum material property value
- mp_max : float; default=1e20
maximum material property value
- validate : bool; default=False
should the variable be validated
- comment : str; default=’‘
a comment for the card
- .. note:: either dvids or labels is required
-
_properties
= ['desvar_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DVMREL2 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
allowed_materials
= ['MAT1', 'MAT2']¶
-
calculate
(self, op2_model, subcase_id)[source]¶ this should really make a call the the DEQATN; see the PBEAM for an example of get/set_opt_value
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
- .. todo:: add support for DEQATN cards to finish DVMREL2 xref
-
mat_type
= None¶ Name of a material entry, such as MAT1, MAT2, etc
-
mid
= None¶ Property entry identification number
-
mp_max
= None¶ Maximum value allowed for this property. (Real; Default = 1.0E20)
-
mp_min
= None¶ Minimum value allowed for this property. If MPNAME references a material property that can only be positive, then the default value for MPMIN is 1.0E-15. Otherwise, it is -1.0E35. (Real)
-
mp_name
= None¶ Property name, such as ‘E’, ‘RHO’ (Character)
-
object_attributes
(self, mode='public', keys_to_skip=None, filter_properties=False)[source]¶ See also
pyNastran.utils.object_attributes(…)
-
type
= 'DVMREL2'¶
-
class
pyNastran.bdf.cards.optimization.
DVPREL1
(oid, prop_type, pid, pname_fid, dvids, coeffs, p_min=None, p_max=1e+20, c0=0.0, validate=False, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.DVXREL1
1 2 3 4 5 6 7 8 DVPREL1 ID TYPE PID PNAME/FID PMIN PMAX C0 DVID1 COEF1 DVID2 COEF2 DVID3 etc. DVPREL1 200000 PCOMP 2000 T2 200000 1.0 Creates a DVPREL1 card
Parameters: - oid : int
optimization id
- prop_type : str
property card name (e.g., PSHELL)
- pid : int
property id
- pname_fid : str/int
optimization parameter as a pname (property name; T) or field number (fid)
- dvids : List[int]
DESVAR ids
- coeffs : List[float]
scale factors for DESVAR ids
- p_min : float; default=None
minimum property value
- p_max : float; default=1e20
maximum property value
- c0 : float; default=0.
offset factor for the variable
- validate : bool; default=False
should the variable be validated
- comment : str; default=’‘
a comment for the card
-
_properties
= ['desvar_ids', 'allowed_properties', 'allowed_elements', 'allowed_masses', 'allowed_properties_mass']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DVPREL1 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
allowed_elements
= ['CELAS2', 'CBAR', 'CBEAM', 'CQUAD4', 'CBUSH', 'CDAMP2']¶
-
allowed_masses
= ['CONM2', 'CMASS2', 'CMASS4']¶
-
allowed_properties
= ['PELAS', 'PROD', 'PTUBE', 'PBAR', 'PBARL', 'PBEAM', 'PBEAML', 'PSHEAR', 'PSHELL', 'PCOMP', 'PCOMPG', 'PBUSH', 'PBUSH1D', 'PGAP', 'PVISC', 'PDAMP', 'PWELD', 'PBMSECT']¶
-
allowed_properties_mass
= ['PMASS']¶
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
-
get_xinit_lower_upper_bound
(self, model)[source]¶ gets the active x value and the lower/upper bounds
-
repr_fields
(self)[source]¶ Gets the fields in their simplified form
Returns: - fields : List[varies]
the fields that define the card
-
type
= 'DVPREL1'¶
-
class
pyNastran.bdf.cards.optimization.
DVPREL2
(oid, prop_type, pid, pname_fid, deqation, dvids=None, labels=None, p_min=None, p_max=1e+20, validate=False, comment='')[source]¶ Bases:
pyNastran.bdf.cards.optimization.DVXREL2
1 2 3 4 5 6 7 8 9 DVPREL2 ID TYPE PID PNAME/FID PMIN PMAX EQID DESVAR DVID1 DVID2 DVID3 DVID4 DVID5 DVID6 DVID7 DVID8 etc. DTABLE LABL1 LABL2 LABL3 LABL4 LABL5 LABL6 LABL7 LABL8 etc. Creates a DVPREL2 card
Parameters: - oid : int
optimization id
- prop_type : str
property card name (e.g., PSHELL)
- pid : int
property id
- pname_fid : str/int
optimization parameter as a pname (property name; T) or field number (fid)
- deqation : int
DEQATN id
- dvids : List[int]; default=None
DESVAR ids
- labels : List[str]; default=None
DTABLE names
- #params : dict[(index, card_type)] = values
#the storage table for the response function #index : int
#a counter
- #card_type : str
#the type of card to pull from #DESVAR, DVPREL1, DRESP2, etc.
- #values : List[int]
#the values for this response
- p_min : float; default=None
minimum property value
- p_max : float; default=1e20
maximum property value
- validate : bool; default=False
should the variable be validated
- comment : str; default=’‘
a comment for the card
- .. note:: either dvids or labels is required
-
_properties
= ['desvar_ids']¶
-
classmethod
add_card
(card, comment='')[source]¶ Adds a DVPREL2 card from
BDF.add_card(...)
Parameters: - card : BDFCard()
a BDFCard object
- comment : str; default=’‘
a comment for the card
-
allowed_elements
= ['CELAS2', 'CBAR', 'CBEAM', 'CQUAD4', 'CBUSH', 'CDAMP2']¶
-
allowed_masses
= ['CONM2', 'CMASS2', 'CMASS4']¶
-
allowed_properties
= ['PELAS', 'PROD', 'PTUBE', 'PBAR', 'PBARL', 'PBEAM', 'PBEAML', 'PSHELL', 'PCOMP', 'PCOMPG', 'PBUSH', 'PBUSH1D', 'PGAP', 'PVISC', 'PDAMP', 'PWELD']¶
-
allowed_properties_mass
= ['PMASS']¶
-
calculate
(self, op2_model, subcase_id)[source]¶ this should really make a call the the DEQATN; see the PBEAM for an example of get/set_optimization_value
-
cross_reference
(self, model)[source]¶ Cross links the card so referenced cards can be extracted directly
Parameters: - model : BDF()
the BDF object
- .. todo:: add support for DEQATN cards to finish DVPREL2 xref
-
get_xinit_lower_upper_bound
(self, model)[source]¶ gets the active x value and the lower/upper bounds
-
p_max
= None¶ Maximum value allowed for this property. (Real; Default = 1.0E20)
-
p_min
= None¶ Minimum value allowed for this property. If FID references a stress recovery location field, then the default value for PMIN is -1.0+35. PMIN must be explicitly set to a negative number for properties that may be less than zero (for example, field ZO on the PCOMP entry). (Real; Default = 1.E-15) .. todo:: bad default (see DVMREL1)
-
pid
= None¶ Property entry identification number
-
pname_fid
= None¶ Property name, such as ‘T’, ‘A’, or field position of the property entry, or word position in the element property table of the analysis model. Property names that begin with an integer such as 12I/T**3 may only be referred to by field position. (Character or Integer 0)
-
prop_type
= None¶ Name of a property entry, such as PBAR, PBEAM, etc
-
type
= 'DVPREL2'¶
-
class
pyNastran.bdf.cards.optimization.
DVXREL1
(oid, dvids, coeffs, c0, comment)[source]¶ Bases:
pyNastran.bdf.cards.base_card.BaseCard
-
desvar_ids
¶
-
-
class
pyNastran.bdf.cards.optimization.
DVXREL2
(oid, dvids, labels, deqation, comment)[source]¶ Bases:
pyNastran.bdf.cards.base_card.BaseCard
-
dequation
= None¶ DEQATN entry identification number. (Integer > 0)
-
desvar_ids
¶
-
oid
= None¶ Unique identification number
-
-
pyNastran.bdf.cards.optimization.
_blank_or_mode
(attb, msg)[source]¶ if it’s blank, it’s a static result, otherwise it’s a mode id
-
pyNastran.bdf.cards.optimization.
_export_dresps_to_hdf5
(h5_file, model, encoding)[source]¶ exports dresps
-
pyNastran.bdf.cards.optimization.
_get_dresp23_table_values
(name, values_list, inline=False)[source]¶ Parameters: - name : str
the name of the response (e.g., DRESP1, DVPREL1)
- values_list : varies
typical : List[int] DNODE : List[List[int], List[int]]
- inline : bool; default=False
used for DNODE
-
pyNastran.bdf.cards.optimization.
_validate_dresp1_force
(property_type, response_type, atta, attb, atti)[source]¶ helper for
validate_dresp
-
pyNastran.bdf.cards.optimization.
_validate_dresp1_stress_strain
(property_type, response_type, atta, attb, atti)[source]¶ helper for
validate_dresp
-
pyNastran.bdf.cards.optimization.
_validate_dresp_property_none
(property_type, response_type, atta, attb, atti)[source]¶ helper for
validate_dresp
-
pyNastran.bdf.cards.optimization.
get_deqatn_args
(dvxrel2, model, desvar_values)[source]¶ gets the arguments for the DEQATN for the DVxREL2
-
pyNastran.bdf.cards.optimization.
get_dvprel_key
(dvprel, prop=None)[source]¶ helper method for the gui
-
pyNastran.bdf.cards.optimization.
get_dvxrel1_coeffs
(dvxrel, model, desvar_values, debug=False)[source]¶ Used by DVPREL1/2, DVMREL1/2, DVCREL1/2, and DVGRID to determine the value for the new property/material/etc. value
-
pyNastran.bdf.cards.optimization.
parse_table_fields
(card_type, card, fields)[source]¶ - params = {
- (0, ‘DRESP1’) = [10, 20], (1, ‘DESVAR’) = [30], (2, ‘DRESP1’) = [40],
}
-
pyNastran.bdf.cards.optimization.
validate_dresp1
(property_type, response_type, atta, attb, atti)[source]¶
-
pyNastran.bdf.cards.optimization.
validate_dvcrel
(validate, element_type, cp_name)[source]¶ Valdiates the DVCREL1/2
Note
words that start with integers (e.g., 12I/T**3) doesn’t support strings